Manage Releases - nx release
Once you have leveraged Nx's powerful code generation and task running capabilities to build your libraries and applications, you will want to share them with your users.
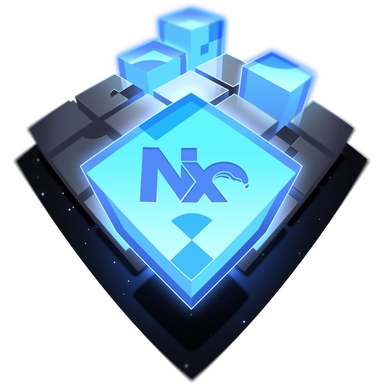
Free Course: Versioning and Releasing NPM packages with Nx
Nx provides a set of tools to help you manage your releases called nx release
.
We recommend always starting with --dry-run, because publishing is difficult to undo
❯
nx release --dry-run
What makes up a release?
A release can be thought about in three main phases:
- Versioning - The process of determining the next version of your projects, and updating any projects that depend on them to use the new version.
- Changelog - The process of deriving a changelog from your commit messages or version plan files, which can be used to communicate the changes to your users.
- Publishing - The process of publishing your projects to a registry, such as npm for TypeScript/JavaScript libraries.
Running releases
The nx release
command is used to run the release process from end to end. It is a wrapper around the three main phases of a release to provide maximum convenience and ease of use.
By default if you just run nx release
it will prompt you for a semver-compatible version number, or semver keyword (such as major, minor, patch, etc.) and then run the three phases of the release process, including publishing.
As with most Nx commands, when trying it out for the first time, it is strongly recommended to use the --dry-run
flag to see what changes will be made before actually making them.
❯
nx release --dry-run
If you are working with a brand new workspace, or one that has never been released before, you will need to establish the previous release before running nx release
. This is because Nx needs to know what the previous version of your projects was in order to know what to use as the start of the new release's changelog commit range. To do this, run git tag
with an appropriate initial version. For example, if you have a brand new workspace, you might run git tag 0.0.0
to establish the initial version.
Customizing releases
The nx release
command is highly customizable. You can customize the versioning, changelog, and publishing phases of the release process independently through a mixture of configuration and CLI arguments.
The configuration lives in your nx.json
file under the release
section.
1{
2 // ... more nx.json config
3 "release": {
4 // For example, configures nx release to target all projects
5 // except the one called "ignore-me"
6 "projects": ["*", "!ignore-me"]
7 // ... nx release config
8 }
9}
10
Customize changelog output
Changelog render options can be passed as an object under release.changelog.projectChangelogs.renderOptions
and release.changelog.workspaceChangelog.renderOptions
in your nx.json
file. Below are all options with their default values for the built-in changelog renderer.
1{
2 // ... more nx.json config
3 "release": {
4 "changelog": {
5 "projectChangelogs": {
6 "renderOptions": {
7 // Whether or not the commit authors should be added to the bottom of the changelog in a "Thank You" section.
8 "authors": true,
9 // Whether or not the commit references (such as commit and/or PR links) should be included in the changelog.
10 "commitReferences": true,
11 // Whether or not to include the date in the version title. It can be set to false to disable it, or true to enable with the default of (YYYY-MM-DD).
12 "versionTitleDate": true,
13 // Whether to apply usernames to authors in the Thank You section. Note, this option was called mapAuthorsToGitHubUsernames prior to Nx v21.
14 "applyUsernameToAuthors": true
15 }
16 },
17 "workspaceChangelog": {
18 "renderOptions": {
19 // Whether or not the commit authors should be added to the bottom of the changelog in a "Thank You" section.
20 "authors": true,
21 // Whether or not the commit references (such as commit and/or PR links) should be included in the changelog.
22 "commitReferences": true,
23 // Whether or not to include the date in the version title. It can be set to false to disable it, or true to enable with the default of (YYYY-MM-DD).
24 "versionTitleDate": true,
25 // Whether to apply usernames to authors in the Thank You section. Note, this option was called mapAuthorsToGitHubUsernames prior to Nx v21.
26 "applyUsernameToAuthors": true
27 }
28 }
29 }
30 }
31}
32
Using nx release subcommands independently
As explained above, nx release
is a wrapper around the three main phases of a release.
If you need more advanced or granular control over your release process you can also run these phases independently using the nx release version
, nx release changelog
, and nx release publish
subcommands.
Each of these subcommands has their own CLI arguments which you can explore using the --help
flag.
❯
nx release version --help
❯
nx release changelog --help
❯
nx release publish --help
Using the programmatic API for nx release
For the maximum control and power over your release process, it is recommended to use the programmatic API for nx release
in your own custom scripts.
Here is a full working example of creating a custom script which processes its own CLI arguments (with --dry-run
true by default) and then calls the nx release
programmatic API.
1import { releaseChangelog, releasePublish, releaseVersion } from 'nx/release';
2import * as yargs from 'yargs';
3
4(async () => {
5 const options = await yargs
6 .version(false) // don't use the default meaning of version in yargs
7 .option('version', {
8 description:
9 'Explicit version specifier to use, if overriding conventional commits',
10 type: 'string',
11 })
12 .option('dryRun', {
13 alias: 'd',
14 description:
15 'Whether or not to perform a dry-run of the release process, defaults to true',
16 type: 'boolean',
17 default: true,
18 })
19 .option('verbose', {
20 description:
21 'Whether or not to enable verbose logging, defaults to false',
22 type: 'boolean',
23 default: false,
24 })
25 .parseAsync();
26
27 const { workspaceVersion, projectsVersionData } = await releaseVersion({
28 specifier: options.version,
29 dryRun: options.dryRun,
30 verbose: options.verbose,
31 });
32
33 await releaseChangelog({
34 versionData: projectsVersionData,
35 version: workspaceVersion,
36 dryRun: options.dryRun,
37 verbose: options.verbose,
38 });
39
40 // publishResults contains a map of project names and their exit codes
41 const publishResults = await releasePublish({
42 dryRun: options.dryRun,
43 verbose: options.verbose,
44 });
45
46 process.exit(
47 Object.values(publishResults).every((result) => result.code === 0) ? 0 : 1
48 );
49})();
50